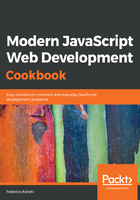
Mapping arrays
A second type of very common operation is to go through an array and produce a new array by doing some kind of process to each element. Fortunately, we also have a way to do that functionally by using .map(). The way this function works is simple: given an array and a function, it applies the function to each element of the array and produces a new array with the results of each call.
Suppose we called a web service and got back an array with people data. We just wanted their ages so that we are able to do some other process; say, calculate the average age of the people who used the service. We can manage this simply:
// Source file: src/map_filter_reduce.js
type person = { name: string, sex: string, age: number };
const family: Array<person> = [
{ name: "Huey", sex: "M", age: 7 },
{ name: "Dewey", sex: "M", age: 8 },
{ name: "Louie", sex: "M", age: 9 },
{ name: "Daisy", sex: "F", age: 25 },
{ name: "Donald", sex: "M", age: 30 },
{ name: "Della", sex: "F", age: 30 }
];
const ages = family.map(x => x.age);
// [7, 8, 9, 25, 30, 30]
Using .map() is, like .reduce(), a much shorter and safer way to process an array. In fact, most times, the two operations are used one after the other, with some possible .filter() operations mixed in to select what should or should not be processed; let's get into that now.