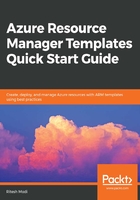
A brief primer on JSON
JavaScript Object Notation (JSON) is a lightweight data-representation and interchange format. It is a text-based and human-readable format that is easy to read, comprehend, and update. Many people think of JSON as a programming language or a markup language; however, it is neither. It is a pure representation of data in text format.
Prior to JSON, XML was the preferred data-exchange format and JSON has few similarities with it. Like XML, JSON is self-describing. It is quite easy to understand the intent of a JSON document by just reading through it. Also, like XML, it can create complex as well as nested objects. It is hierarchical, and objects can be composed of child objects and arrays. However, the similarities end here. JSON documents are terser and much more compact compared to XML documents. As a result, they are also much more reader-friendly. The data in XML documents is all string-based, however, in JSON, data can have types that make it easier to parse and validate them. The XML syntax is quite verbose compared to JSON.
JSON is supported by almost all programming languages and it provides SDKs to generate as well as read them intuitively.
There are a few limitations when it comes to JSON documents. They do not have any schema definition and documents do not have any fixed specification associated to them. In effect, there are no parsers or facilities to validate that a JSON document adheres to a certain specification.
A simple JSON document is shown here:
“customer” : {
“customerId”: 1,
“firstName”: “ritesh”,
“lastName”: “Modi”,
“isRepeatCustomer”: true,
“address”: {
“streetName”: “10, Park Street”,
“city”: “Mumbai”,
“Country”: “India”
},
“productsOrdered”: [“IPhone”, “TShirt”]
}
Readers will notice that this JSON document is quite easy to read and comprehend. The basic JSON syntax comprises name-value pairs, where the name part is always decorated in double-quotes. Also, as a practice, the name part of the name-value pair follows the camel-casing naming standard. Each name is followed by a colon, :, and subsequently followed by the value part. Each name-value pair is separated using a comma: ,.
A JSON document starts with a left curly bracket, {, and ends with a right curly bracket: }. A JSON value can hold values of numbers, strings, Boolean, objects, and array data types:
- Strings: Strings are a sequence of continuous characters and always enclosed within double quotes. In the preceding example, India is a string value assigned to Country. "Country": "India"; since double-quotes are special characters in JSON, they need to be escaped if they are part of the value. For example: "/"India/"" will have an output of "India" assigned to the name element instead of just India without quotes.
- Numbers: JSON supports multiple data types related to numbers, including integers, scientific, and real numbers.
- Boolean: JSON supports true and false as Boolean values. They are never enclosed within quotes.
- Null: JSON also supports Null as a value. It means nothing is assigned to the name element.
- Objects: JSON also has a concept of an object. An object is a collection of key-value pairs enclosed within left curly brackets, {, and right curly brackets, }. Objects can be nested; that is, an object can contain another object as a child object. The customer document shown before in this section is an example of a JSON object.
- Arrays: JSON arrays start with left square brackets, [, and end with right square brackets, ]. JSON arrays contain name-value pairs separated by a comma. They can also contain a set of values. productsOrdered in the previous example was an example of JSON arrays.
ARM templates support the following additional objects:
- SecureString: A secure string is similar to a native JSON string; the difference is that the ARM templates runtime ensures that these values are never written in log files. These values are not visible on the Azure portal. This datatype is used to pass credentials, keys, and secrets that should not be visible to anyone.
- SecureObject: A secure object is similar to native JSON arrays and objects. Again, these values are not visible in Azure portal and log files and are used to pass arrays and objects as secrets to ARM templates.