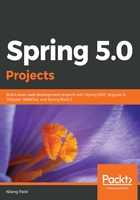
Defining the data access layer – Spring JDBC Template
We have the model classes that reflect the structure of the data in the database that we obtained from the World Bank API. Now we need to develop a data access layer that interacts with our MySQL and populates the data stored in the database into instances of the model classes. We will use the Spring JDBC Template to achieve the required interaction with the database.
First, we need the JDBC driver to connect any Java application with MySQL. This can be obtained by adding the following dependency and version property to our pom.xml:
<properties>
<java.version>1.8</java.version>
<lombok.version>1.16.18</lombok.version>
<hibernate.validator.version>6.0.2.Final</hibernate.validator.version>
<mysql.jdbc.driver.version>5.1.44</mysql.jdbc.driver.version>
</properties>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>${mysql.jdbc.driver.version}</version>
</dependency>
Anything that comes as <dependency></dependency> goes within the <dependencies></dependencies> list.
Now we need to add a dependency to the Spring core APIs, as well as the Spring JDBC APIs (which contain the JDBC Template) to our pom.xml. A brief intro about these two dependencies is as follows:
- Spring core APIs: It provides us with core Spring features such as dependency injection and configuration model
- Spring JDBC APIs: It provides us with the APIs required to create the DataSource instance and interact with the database
The following code shows the dependency information for the two libraries:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.version}</version>
</dependency>
Along with the preceding two dependencies, we need to add a few more Spring dependencies to assist us in setting up Java-based configurations using annotations (such as @bean, @Service, @Configuration, @ComponentScan, and so on) and dependency injection using annotations (@Autowired). For this, we will be adding further dependencies as follows:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-beans</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
</dependency>