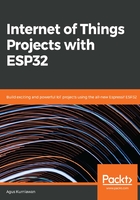
上QQ阅读APP看书,第一时间看更新
Writing an ESP32 program
To write our program, we need to follow these steps:
- Modify the tft_demo.c file from the TFT library for ESP32. For this, we will use the circle_demo() function, as written here:
static void circle_demo()
{
int x, y, r, n;
//In the upper part of the LCD display print the message “CIRCLE DEMO
disp_header("CIRCLE DEMO");
- Now draw some random circles on the LCD display using the TFT_drawCircle function. The circles will be randomly positioned on the screen and will have a random color with the help of the function random_color(). After that, the bottom header will be updated with the number of drawn circles (for example, 208 CIRCLES).
uint32_t end_time = clock() + GDEMO_TIME;
n = 0;
while ((clock() < end_time) && (Wait(0))) {
x = rand_interval(8, dispWin.x2-8);
y = rand_interval(8, dispWin.y2-8);
if (x < y) r = rand_interval(2, x/2);
else r = rand_interval(2, y/2);
TFT_drawCircle(x,y,r,random_color());
n++;
}
sprintf(tmp_buff, "%d CIRCLES", n);
update_header(NULL, tmp_buff);
Wait(-GDEMO_INFO_TIME);
- Now is the time to not only draw some circles but also fill to them with colors using the function TFT_fillCircle(). In the end, using the same values for x, y and r but different random_color() values, the circles will have a different filling and a different edge color.
update_header("FILLED CIRCLE", "");
TFT_fillWindow(TFT_BLACK);
end_time = clock() + GDEMO_TIME;
n = 0;
while ((clock() < end_time) && (Wait(0))) {
x = rand_interval(8, dispWin.x2-8);
y = rand_interval(8, dispWin.y2-8);
if (x < y) r = rand_interval(2, x/2);
else r = rand_interval(2, y/2);
TFT_fillCircle(x,y,r,random_color());
TFT_drawCircle(x,y,r,random_color());
n++;
}
sprintf(tmp_buff, "%d CIRCLES", n);
update_header(NULL, tmp_buff);
Wait(-GDEMO_INFO_TIME);
}
- Next, modify the tft_demo() function that calls the circle_demo() function:
void tft_demo() {
...
// demo
disp_header("Welcome to ESP32");
circle_demo();
while (1) {
// do nothing
}
}
- Since we will use some components that are located on <project>/components, we should tell the compiler to include these components. We will add the component.mk file with our components included, as follows:
COMPONENT_SRCDIRS := .
COMPONENT_ADD_INCLUDEDIRS := .
- For the Makefile file, we can write these scripts as follows:
PROJECT_NAME := lcddemo
EXTRA_CFLAGS += --save-temps
include $(IDF_PATH)/make/project.mk
- Save all files before configuring our project to compile and flash our program into the ESP32 board.
Now that we've created the program, let's configure our board.