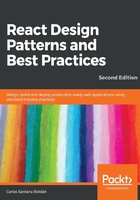
上QQ阅读APP看书,第一时间看更新
Airbnb React/JSX style guide
The Airbnb React/JSX style guide is the most popular style guide for coding in React.
To implement the Airbnb React/JSX style guide, we need to install some packages:
npm install --save-dev babel-eslint eslint eslint-config-airbnb eslint-plugin-import eslint-plugin-jsx-a11y eslint-plugin-react
You can check all the ESLint rules on the official website (https://eslint.org/docs/rules) and all the special React ESLint rules at https://github.com/yannickcr/eslint-plugin-react/tree/master/docs/rules.
The rules that I prefer not to use, or that I prefer to change the default values of, are as follows:
- comma-dangle: Off
- arrow-parens: Off
- max-len: 120
- no-param-reassign: Off
- function-paren-newline: Off
- react/require-default-props: Off
First, you need to create a new file called .eslintrc at the root level:
{
"parser": "babel-eslint",
"extends": "airbnb",
"rules": {
"arrow-parens": "off",
"comma-dangle": "off",
"function-paren-newline": "off",
"max-len": [1, 120],
"no-param-reassign": "off",
"react/require-default-props": "off"
}
}
To run your linter, you have to add a lint script into your package.json file:
{
"scripts": {
"lint": "eslint --ext .jsx,.js src"
}
}
Now you can lint your files using the Airbnb React/JSX style guide.