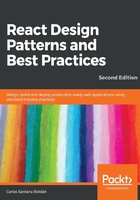
上QQ阅读APP看书,第一时间看更新
Root
One important difference with HTML worth mentioning is that, since JSX elements get translated into JavaScript functions, and you cannot return two functions in JavaScript, whenever you have multiple elements at the same level you are forced to wrap them into a parent.
Let's look at a simple example:
<div />
<div />
This gives us the following error:
Adjacent JSX elements must be wrapped in an enclosing tag.
On the other hand, the following works:
<div>
<div />
<div />
</div>
Before, React forced you to return an element wrapped with a <div> element or any other tag; since React 16.2.0, it is possible to return an array or string directly:
// Example 1: Returning an array of elements.
render() {
// Now you don't need to wrap list items in an extra element
return [
<li key="1">First item</li>,
<li key="2">Second item</li>,
<li key="3">Third item</li>
];
}
// Example 2: Returning a string
render() {
return 'Hello World!';
}
Also, React now has a new feature called Fragment that also works as a special wrapper for elements. It can be specified with empty tags (<></>) or directly using React.Fragment:
// Example 1: Using empty tags <></>
render() {
return (
<>
<ComponentA />
<ComponentB />
<ComponentC />
</>
);
}
// Example 2: Using React.Fragment
render() {
return (
<React.Fragment>
<h1>An h1 heading</h1>
Some text here.
<h2>An h2 heading</h2>
More text here.
Even more text here.
</React.Fragment>
);
}
// Example 3: Importing Fragment
import React, { Fragment } from 'react';
render() {
return (
<Fragment>
<h1>An h1 heading</h1>
Some text here.
<h2>An h2 heading</h2>
More text here.
Even more text here.
</Fragment>
);
}