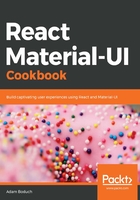
How it works...
Let's start by looking at the Route components that render content based on the active the Route components:
<Grid item className={classes.alignContent}>
<Route
exact
path="/"
render={() => <Typography>Home</Typography>}
/>
<Route
exact
path="/page2"
render={() => <Typography>Page 2</Typography>}
/>
<Route
exact
path="/page3"
render={() => <Typography>Page 3</Typography>}
/>
</Grid>
There's a Route component used for each path in your app. The render() function returns the content that should be rendered within this Grid item when the path property matches the current URL.
Next, let's look at one of the ListItem components within the Drawer component, as follows:
<ListItem
component={Link}
to="/"
onClick={() => setOpen(false)}
>
<ListItemIcon>
<HomeIcon />
</ListItemIcon>
<ListItemText>Home</ListItemText>
</ListItem>
By default, the ListItem component will render a div element. It accepts a button property that when true, will render a button element. You don't want either of these. Instead, you want the list items to be links that react-router will process. The component property accepts a custom component to use; in this example, you want to use the Link component from the react-router-dom package. This will render the appropriate link while maintaining the proper styles.
The properties that you pass to ListItem are also passed to your custom component, which, in this case, is Link. This means that the required to property is passed to Link, pointing the link to /. Likewise, the onClick handler is also passed to the Link component, which is important because you want to close the temporary drawer whenever a link is clicked.