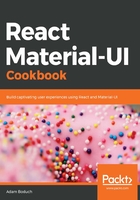
上QQ阅读APP看书,第一时间看更新
How to do it...
To hide the AppBar component while the user is scrolling down, you have to know when the user is scrolling. This requires listening to the scroll event on the window object. You can implement a component that listens to this event and hides the AppBar component while scrolling. Here's how it's done:
import React, { Component } from 'react';
import { withStyles } from '@material-ui/core/styles';
import AppBar from '@material-ui/core/AppBar';
import Toolbar from '@material-ui/core/Toolbar';
import Typography from '@material-ui/core/Typography';
import Button from '@material-ui/core/Button';
import IconButton from '@material-ui/core/IconButton';
import MenuIcon from '@material-ui/icons/Menu';
import Fade from '@material-ui/core/Fade';
const styles = theme => ({
root: {
flexGrow: 1
},
flex: {
flex: 1
},
menuButton: {
marginLeft: -12,
marginRight: 20
},
toolbarMargin: theme.mixins.toolbar
});
const ScrolledAppBar = withStyles(styles)(
class extends Component {
state = {
scrolling: false,
scrollTop: 0
};
onScroll = e => {
this.setState(state => ({
scrollTop: e.target.documentElement.scrollTop,
scrolling:
e.target.documentElement.scrollTop > state.scrollTop
}));
};
shouldComponentUpdate(props, state) {
return this.state.scrolling !== state.scrolling;
}
componentDidMount() {
window.addEventListener('scroll', this.onScroll);
}
componentWillUnmount() {
window.removeEventListener('scroll', this.onScroll);
}
render() {
const { classes } = this.props;
return (
<Fade in={!this.state.scrolling}>
<AppBar>
<Toolbar>
<IconButton
className={classes.menuButton}
color="inherit"
aria-label="Menu"
>
<MenuIcon />
</IconButton>
<Typography
variant="h6"
color="inherit"
className={classes.flex}
>
My Title
</Typography>
<Button color="inherit">Login</Button>
</Toolbar>
</AppBar>
</Fade>
);
}
}
);
const AppBarWithButtons = withStyles(styles)(
({ classes, title, buttonText }) => (
<div className={classes.root}>
<ScrolledAppBar />
<div className={classes.toolbarMargin} />
<ul>
{new Array(500).fill(null).map((v, i) => (
<li key={i}>{i}</li>
))}
</ul>
</div>
)
);
export default AppBarWithButtons;
When you first load the screen, the toolbar and content appear as usual:

When you scroll down, the AppBar component disappears, allowing more space for the content to be viewed. Here's what the screen looks like when you scroll to the very bottom:

The AppBar component will reappear as soon as you start scrolling back up.