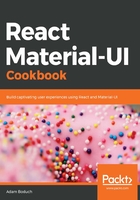
There's more...
When the screen in this example first loads, some of the content is hidden behind the AppBar component. This is because the position is fixed and it has a higher z-index value than the regular content. This is expected, so that when you scroll, the regular content goes behind the AppBar component. The solution is to add a top margin to your content. The problem is that you don't necessarily know the height of the AppBar.
You could just set a value that looks good. A better solution is to use the toolbar mixin styles. You can access this mixin object by making styles a function that returns an object. Then, you'll have access to the theme argument, which has a toolbar mixin object.
Here's what styles should be changed to:
const styles = theme => ({
root: {
flexGrow: 1
},
flex: {
flex: 1
},
menuButton: {
marginLeft: -12,
marginRight: 20
},
toolbarMargin: theme.mixins.toolbar
});
The new style that's added is toolbarMargin. Notice that this is using the value from theme.mixins.toolbar, which is why you're using a function now – so that you can access theme. Here's what the theme.mixins.toolbar value looks like:
{
"minHeight": 56,
"@media (min-width:0px) and (orientation: landscape)": {
"minHeight": 48
},
"@media (min-width:600px)": {
"minHeight": 64
}
}
The last step is to add a <div> element to the content underneath the AppBar component where this new toolbarMargin style can be applied:
<div className={classes.root}>
<AppBar position="fixed">
<Toolbar>
<IconButton
className={classes.menuButton}
color="inherit"
aria-label="Menu"
>
<MenuIcon />
</IconButton>
<Typography
variant="title"
color="inherit"
className={classes.flex}
>
Title
</Typography>
<Button color="inherit">Login</Button>
</Toolbar>
</AppBar>
<div className={classes.toolbarMargin} />
<ul>
{new Array(500).fill(null).map((v, i) => <li key={i}>{i}</li>)}
</ul>
</div>
Now, the beginning of the content is no longer hidden by the AppBar component when the screen first loads:
