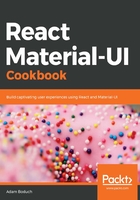
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's say that you have a four-column layout, but you want the first and second items to go in the first column, the third and fourth items in the second, and so on. This involves using nested Grid containers, and changing the direction property, as follows:
import React from 'react';
import { withStyles } from '@material-ui/core/styles';
import Paper from '@material-ui/core/Paper';
import Grid from '@material-ui/core/Grid';
import Hidden from '@material-ui/core/Hidden';
import Typography from '@material-ui/core/Typography';
const styles = theme => ({
root: {
flexGrow: 1
},
paper: {
padding: theme.spacing(2),
textAlign: 'center',
color: theme.palette.text.secondary
}
});
const ColumnDirection = withStyles(styles)(({ classes }) => (
<div className={classes.root}>
<Grid container justify="space-around" spacing={4}>
<Grid item xs={3}>
<Grid container direction="column" spacing={2}>
<Grid item>
<Paper className={classes.paper}>
<Typography>One</Typography>
</Paper>
</Grid>
<Grid item>
<Paper className={classes.paper}>
<Typography>Two</Typography>
</Paper>
</Grid>
</Grid>
</Grid>
<Grid item xs={3}>
<Grid container direction="column" spacing={2}>
<Grid item>
<Paper className={classes.paper}>
<Typography>Three</Typography>
</Paper>
</Grid>
<Grid item>
<Paper className={classes.paper}>
<Typography>Four</Typography>
</Paper>
</Grid>
</Grid>
</Grid>
<Grid item xs={3}>
<Grid container direction="column" spacing={2}>
<Grid item>
<Paper className={classes.paper}>
<Typography>Five</Typography>
</Paper>
</Grid>
<Grid item>
<Paper className={classes.paper}>
<Typography>Six</Typography>
</Paper>
</Grid>
</Grid>
</Grid>
<Grid item xs={3}>
<Grid container direction="column" spacing={2}>
<Grid item>
<Paper className={classes.paper}>
<Typography>Seven</Typography>
</Paper>
</Grid>
<Grid item>
<Paper className={classes.paper}>
<Typography>Eight</Typography>
</Paper>
</Grid>
</Grid>
</Grid>
</Grid>
</div>
));
export default ColumnDirection;
Here's what the result looks like at a pixel width of 725:

Instead of values flowing from left to right, you have complete control over which column the item is placed in.
You might have noticed that the font looks different, compared to other examples in this chapter. This is because of the Typography component used to style the text and apply Material-UI theme styles. Most Material-UI components that display text don't require you to use Typography, but Paper does.