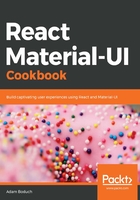
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's say that you have eight Paper components that you want to render, but you also want to make sure that there are no more than four columns. Use the following code to do this:
import React from 'react';
import { withStyles } from '@material-ui/core/styles';
import Paper from '@material-ui/core/Paper';
import Grid from '@material-ui/core/Grid';
const styles = theme => ({
root: {
flexGrow: 1
},
paper: {
padding: theme.spacing(2),
textAlign: 'center',
color: theme.palette.text.secondary
}
});
const FixedColumnLayout = withStyles(styles)(({ classes, width }) => (
<div className={classes.root}>
<Grid container spacing={4}>
<Grid item xs={width}>
<Paper className={classes.paper}>xs={width}</Paper>
</Grid>
<Grid item xs={width}>
<Paper className={classes.paper}>xs={width}</Paper>
</Grid>
<Grid item xs={width}>
<Paper className={classes.paper}>xs={width}</Paper>
</Grid>
<Grid item xs={width}>
<Paper className={classes.paper}>xs={width}</Paper>
</Grid>
<Grid item xs={width}>
<Paper className={classes.paper}>xs={width}</Paper>
</Grid>
<Grid item xs={width}>
<Paper className={classes.paper}>xs={width}</Paper>
</Grid>
<Grid item xs={width}>
<Paper className={classes.paper}>xs={width}</Paper>
</Grid>
<Grid item xs={width}>
<Paper className={classes.paper}>xs={width}</Paper>
</Grid>
</Grid>
</div>
));
export default FixedColumnLayout;
Here's what the result looks like with a pixel width of 725:

Here's what the result looks like with a pixel width of 350:
