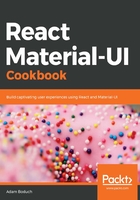
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's say that you have four elements that you want to lay out on the screen so that they're evenly spaced and occupy all available horizontal space. The code for this is as follows:
import React from 'react';
import { withStyles } from '@material-ui/core/styles';
import Paper from '@material-ui/core/Paper';
import Grid from '@material-ui/core/Grid';
const styles = theme => ({
root: {
flexGrow: 1
},
paper: {
padding: theme.spacing(2),
textAlign: 'center',
color: theme.palette.text.secondary
}
});
const UnderstandingBreakpoints = withStyles(styles)(({ classes }) => (
<div className={classes.root}>
<Grid container spacing={4}>
<Grid item xs={12} sm={6} md={3}>
<Paper className={classes.paper}>xs=12 sm=6 md=3</Paper>
</Grid>
<Grid item xs={12} sm={6} md={3}>
<Paper className={classes.paper}>xs=12 sm=6 md=3</Paper>
</Grid>
<Grid item xs={12} sm={6} md={3}>
<Paper className={classes.paper}>xs=12 sm=6 md=3</Paper>
</Grid>
<Grid item xs={12} sm={6} md={3}>
<Paper className={classes.paper}>xs=12 sm=6 md=3</Paper>
</Grid>
</Grid>
</div>
));
export default UnderstandingBreakpoints;
This renders four Paper components. The labels indicate the values used for the xs, sm, and md properties. Here's what the result looks like:
