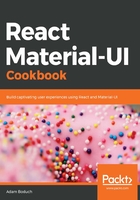
There's more...
The centered layout for tabs works well on smaller screens, while the full width layout looks good on larger screens. You can use Material-UI utilities that tell you about breakpoint changes. You can then use this information to change the alignment of your tabs.
Here's a modified version of this example:
import React, { useState } from 'react';
import compose from 'recompose/compose';
import { withStyles } from '@material-ui/core/styles';
import withWidth from '@material-ui/core/withWidth';
import Tabs from '@material-ui/core/Tabs';
import Tab from '@material-ui/core/Tab';
const styles = theme => ({
root: {
flexGrow: 1,
backgroundColor: theme.palette.background.paper
}
});
function TabAlignment({ classes, width }) {
const [value, setValue] = useState(0);
const onChange = (e, value) => {
setValue(value);
};
return (
<div className={classes.root}>
<Tabs
value={value}
onChange={onChange}
variant={['xs', 'sm'].includes(width) ? null : 'fullWidth'}
centered
>
<Tab label="Item One" />
<Tab label="Item Two" />
<Tab label="Item Three" />
</Tabs>
</div>
);
}
export default compose(
withWidth(),
withStyles(styles)
)(TabAlignment);
Now when you resize your screen, the alignment properties of the grid can change in response to breakpoint changes. Let's break down these changes from the bottom up, starting with the variant property value:
variant={['xs', 'sm'].includes(width) ? null : 'fullWidth'}
The value will be fullWidth if the width property is anything but the xs or sm breakpoint. In other words, if it's a larger screen, the value will be fullWidth.
Next, you need the width property to be passed to your component somehow. You can use the withWidth() utility from Material-UI. It works like withStyles() in that it returns a new component with new properties assigned to it. The component returned by withWidth() will update its width prop any time the breakpoint changes. For example, if the user resizes their screen from sm to md, this will trigger a width change and fullWidth will change from false to true.
To use the withWidth() component—along with the withStyles() component—you can use the compose() function from recompose. This function makes your code more readable when you're applying several higher-order functions that decorate your component:
export default compose(
withWidth(),
withStyles(styles)
)(TabAlignment);
You could call withWidth(withStyles(styles))(TabAlignment) if you really don't want to use recompose, but as a general rule, I like to use it any time more than one higher-order function is involved.