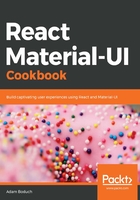
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's say that you have a Tabs component with three Tab buttons. Instead of rendering the tabs so that they look as though they're floating on the screen, you can wrap them in an AppBar component to give them a contained look and feel. Here's the code:
import React, { useState } from 'react';
import { withStyles } from '@material-ui/core/styles';
import AppBar from '@material-ui/core/AppBar';
import Tabs from '@material-ui/core/Tabs';
import Tab from '@material-ui/core/Tab';
import Typography from '@material-ui/core/Typography';
const styles = theme => ({
root: {
flexGrow: 1,
backgroundColor: theme.palette.background.paper
},
tabContent: {
padding: theme.spacing.unit * 2
}
});
function AppBarIntegration({ classes }) {
const [value, setValue] = useState(0);
const onChange = (e, value) => {
setValue(value);
};
return (
<div className={classes.root}>
<AppBar position="static">
<Tabs value={value} onChange={onChange}>
<Tab label="Item One" />
<Tab label="Item Two" />
<Tab label="Item Three" />
</Tabs>
</AppBar>
{value === 0 && (
<Typography component="div" className={classes.tabContent}>
Item One
</Typography>
)}
{value === 1 && (
<Typography component="div" className={classes.tabContent}>
Item Two
</Typography>
)}
{value === 2 && (
<Typography component="div" className={classes.tabContent}>
Item Three
</Typography>
)}
</div>
);
}
export default withStyles(styles)(AppBarIntegration);
When the screen first loads, you'll see the following:

When you click on one of the tab buttons, the selected tab changes, along with the content underneath the tabs. For example, clicking on the ITEM THREE tab results in this:
