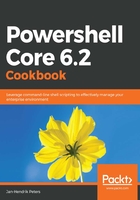
上QQ阅读APP看书,第一时间看更新
How to do it...
Perform the following steps:
- Let's start with a seemingly simple static method, TryParse, in the Boolean class:
[bool] | Get-Member -Static -Name TryParse
- To be able to use this method, the parameter modifier [out] is needed. [Out] indicates that a parameter value is passed as a reference that will be used as output, for example, to a variable:
$parsedValue = $null
$parseSuccess = [bool]::TryParse('False', [ref]$parsedValue)
Write-Host "Parsing 'False' to boolean was successful: $parsesuccess. The parsed boolean is $parsedValue"
- The output of Get-Member is a bit misleading. While a reference is indeed passed, [out] and [ref] are not the same:
From https://docs.microsoft.com/en-us/dotnet/api/system.boolean.tryparse?view=netcore-2.2:
public static bool TryParse (string value, out bool result);
The keyword ref indicates that a reference to the heap is passed as a function parameter instead of the actual value itself.
- Ref can be used in PowerShell as the data type of a cmdlet parameter, enabling you to use [ref] in PowerShell as well:
# [ref] in PowerShell
function ByReference
{
param(
[ref]
$ReferenceObject
)
$ReferenceObject.Value = 42
}
$valueType = 7
ByReference -ReferenceObject ([ref]$valueType)
$valueType.GetType() # Still a value type, but the value has been changed
- In C#, developers also regularly use delegate methods. Most prominently, they are used with LINQ, which are language-integrated queries:
# Delegate methods like Actions and Funcs are often used in C#, but you can also use them in PowerShell
# The LINQ Where method in C# looks like this:
# processes.Where(proc => proc.WorkingSet64 > 150*1024);
# The proper type cast is important. The output of Get-Process is normally an Object array!
[System.Diagnostics.Process[]]$procs = Get-Process
# The delegate type that LINQ expects is a Func. This type expects two
# parameters, a Type parameter indicating the source data type, e.g. Process as well as a predicate, the filter
[Func[System.Diagnostics.Process,bool]] $delegate = { param($proc); return $proc.WorkingSet64 -gt 150mb }
[Linq.Enumerable]::Where($procs, $delegate)
# The same delegate can be used with e.g. First, which filters like where and returns the first object
# matching the filter
[Linq.Enumerable]::First($procs, $delegate)