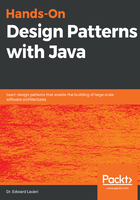
上QQ阅读APP看书,第一时间看更新
Accessors and mutators
Accessor methods are those that allow an object's data to be accessed. These methods can get the data, but not change it. This is a great way to protect the data from being changed. Accessor methods are also referred to as Getters methods.
Mutator methods, also known as setter methods, allow the object's instance variables to be changed.
Here is a complete set of accessors and mutators for our Bicycle class:
// Accessors (Getters)
public int getGears() {
return this.gears;
}
public double getCost() {
return this.cost;
}
public double getWeight() {
return this.weight;
}
public String getColor() {
return this.color;
}
// Mutators (Setters)
public void setGears(int nbr) {
this.gears = nbr;
}
public void setCost(double amt) {
this.cost = amt;
}
public void setWeight(double lbs) {
this.weight = lbs;
}
public void setColor(String theColor) {
this.color = theColor;
}
As you can see in the preceding code, we use the this reference for the current object. The accessors do not take any parameters and simply return the value of the instance variable. The mutators take a parameter and use its value in assigning a new value to an instance variable.