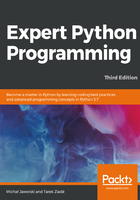
Other idioms
Another typical example of a Python idiom is the use of enumerate(). This built-in function provides a convenient way to get an index when a sequence is iterated inside of a loop. Consider the following piece of code as an example of tracking the element index without the enumerate() function:
>>> i = 0 >>> for element in ['one', 'two', 'three']: ... print(i, element) ... i += 1 ... 0 one 1 two 2 three
This can be replaced with the following code, which is shorter and definitely cleaner:
>>> for i, element in enumerate(['one', 'two', 'three']): ... print(i, element) ... 0 one 1 two 2 three
If you need to aggregate elements of multiple lists (or any other iterables) in the one-by-one fashion, you can use the built-in zip(). This is a very common pattern for uniform iteration over two same-sized iterables:
>>> for items in zip([1, 2, 3], [4, 5, 6]): ... print(items) ... (1, 4) (2, 5) (3, 6)
Note that the results of zip() can be reversed by another zip() call:
>>> for items in zip(*zip([1, 2, 3], [4, 5, 6])): ... print(items) ... (1, 2, 3) (4, 5, 6)
One important thing you need to remember about the zip() function is that it expects input iterables to be the same size. If you provide arguments of different lengths, then it will trim the output to the shortest argument, as shown in the following example:
>>> for items in zip([1, 2, 3, 4], [1, 2]):
... print(items)
...
(1, 1)
(2, 2)
Another popular syntax element is sequence unpacking. It is not limited to lists and tuples, and will work with any sequence type (even strings and byte sequences). It allows us to unpack a sequence of elements into another set of variables as long as there are as many variables on the left-hand side of the assignment operator as the number of elements in the sequence. If you paid attention to the code snippets, then you might have already noticed this idiom when we were discussing the enumarate() function.
The following is a dedicated example of that syntax element:
>>> first, second, third = "foo", "bar", 100 >>> first 'foo' >>> second 'bar' >>> third 100
Unpacking also allows us to capture multiple elements in a single variable using starred expressions as long as it can be interpreted unambiguously. Unpacking can also be performed on nested sequences. This can come in handy, especially when iterating on some complex data structures built out of multiple sequences. Here are some examples of more complex sequence unpacking:
>>> # starred expression to capture rest of the sequence >>> first, second, *rest = 0, 1, 2, 3 >>> first 0 >>> second 1 >>> rest [2, 3] >>> # starred expression to capture middle of the sequence >>> first, *inner, last = 0, 1, 2, 3 >>> first 0 >>> inner [1, 2] >>> last 3 >>> # nested unpacking >>> (a, b), (c, d) = (1, 2), (3, 4) >>> a, b, c, d (1, 2, 3, 4)