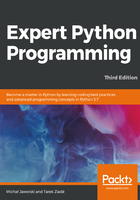
Interactive debuggers
Code debugging is an integral element of the software development process. Many programmers can spend most of their life using only extensive logging and print statements as their primary debugging tools, but most professional developers prefer to rely on some kind of debugger.
Python already ships with a built-in interactive debugger called pdb (refer to https://docs.python.org/3/library/pdb.html). It can be invoked from the command line on the existing script, so Python will enter post-mortem debugging if the program exits abnormally:
python -m pdb script.py
Post-mortem debugging, while useful, does not cover every scenario. It is useful only when the application exits with some exception if the bug occurs. In many cases, faulty code just behaves abnormally, but does not exit unexpectedly. In such cases, custom breakpoints can be set on a specific line of code using this single-line idiom:
import pdb; pdb.set_trace()
This will cause the Python interpreter to start the debugger session on this line during runtime.
pdb is very useful for tracing issues, and at first glance it may look very familiar to the well-known GNU Debugger (GDB). Because Python is a dynamic language, the pdb session is very similar to an ordinary interpreter session. This means that the developer is not limited to tracing code execution, but can call any code and even perform module imports.
Sadly, because of its roots (bdb), your first experience with pdb can be a bit overwhelming due to the existence of cryptic short-letter debugger commands such as h, b, s, n, j, and r. When in doubt, the help pdb command which can be typed during the debugger session, will provide extensive usage and additional information.
The debugger session in pdb is also very simple and does not provide additional features such as tab completion or code highlighting. Fortunately, there are a few packages available on PyPI that provide such features from alternative Python shells, as mentioned in the previous section. The most notable examples are as follows:
- ipdb: This is a separate package based on ipython
- ptpdb: This is a separate package based on ptpython
- bpdb: This is bundled with bpython