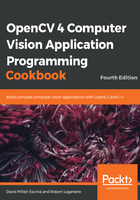
Overloaded image operators
Very conveniently, most arithmetic functions have their corresponding operator overloaded in OpenCV 2. Consequently, the call to cv::addWeighted can be written as follows:
result= 0.7*image1+0.9*image2;
The preceding code is a more compact form that is also easier to read. These two ways of writing the weighted sum are equivalent. In particular, the cv::saturate_cast function will still be called in both cases.
Most C++ operators have been overloaded. Among them are the bitwise operators &, |, ^, and ~; the min, max, and abs functions. The comparison operators <, <=, ==, !=, >, and >= have also been overloaded, and they return an 8-bit binary image. You will also find the m1*m2 matrix multiplication (where m1 and m2 are both cv::Mat instances), the m1.inv() matrix inversion, the m1.t() transpose, the m1.determinant() determinant, the v1.norm() vector norm, the v1.cross(v2) cross-product, the v1.dot(v2) dot product, and so on. When this makes sense, you also have the corresponding operator/assignment operator defined (the += operator, for example).
In the Writing efficient image-scanning loops recipe, we presented a color reduction function that was written using loops that scan the image pixels to perform some arithmetic operations on them. From what we learned here, this function could be rewritten simply using arithmetic operators on the input image as follows:
image=(image&cv::Scalar(mask,mask,mask)) +cv::Scalar(div/2,div/2,div/2);
The use of cv::Scalar is due to the fact that we are manipulating a color image. Performing the same test as we did in the Writing efficient image-scanning loops recipe, we obtain an execution time of 53 ms. Using the image operators makes the code so simple, and the programmer so productive, that you should consider their use in most situations.