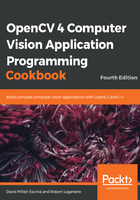
上QQ阅读APP看书,第一时间看更新
How to do it...
To create the sharpen function, we are going to follow these steps:
- We are going to create a sharpen function with an input and output image. This time, the processing cannot be accomplished in-place; the users need to provide an output image:
void sharpen(const cv::Mat &image, cv::Mat &result) {
- Allocate the output result image and get the number of channels of the input image with the .channels() function:
// allocate if necessary result.create(image.size(), image.type()); int nchannels= image.channels(); // get number of channels
- We have to loop for every row. The image scanning is done using three pointers, one for the current line, one for the preceding line, and another one for the following line. Also, since each pixel computation requires access to its neighbors, it is not possible to compute a value for the pixels of the first and last rows of the image as well as the pixels of the first and last columns. The loop can then be written as follows:
// for all rows (except first and last) for (int j= 1; j<image.rows-1; j++) { const uchar* previous= image.ptr<const uchar>(j-1); // previous row const uchar* current= image.ptr<const uchar>(j); // current row const uchar* next= image.ptr<const uchar>(j+1); // next row uchar* output= result.ptr<uchar>(j); // output row for (int i=nchannels; i<(image.cols-1)*nchannels; i++) { *output++= cv::saturate_cast<uchar>( 5*current[i]-current[i-nchannels]- current[i+nchannels]-previous[i]-next[i]); } } }
Note how we wrote the function so that it would work on both gray-level and color images. If we apply this function on a gray-level version of our test image, the following result is obtained:

Let's see how the instructions work when we execute them.