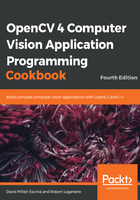
The functor or function object
Using the C++ operator overloading, it is possible to create a class for which its instances behave like functions. The idea is to overload the operator() method, such that a call to the processing method of a class behaves exactly like a simple function call. The resulting class instance is called a function object, or a functor. Often, a functor includes a full constructor, such that it can be used immediately after being created. For example, you can add the following constructor to your ColorDetector class:
// full constructor ColorDetector(uchar blue, uchar green, uchar red, int maxDist=100): maxDist(maxDist) { // target color setTargetColor(blue, green, red); }
Obviously, you can still use the setters and getters that were defined previously. The functor method can be defined as follows:
cv::Mat operator()(const cv::Mat &image) { // color detection code here ... }
To detect a given color with this functor method, simply write the following code snippet:
ColorDetector colordetector(230,190,130, // color 100); // threshold cv::Mat result= colordetector(image); // functor call
As you can see, the call to the color detection method now looks like a function call. As a matter of fact, the colordetector variable can be used as if it were the name of a function.