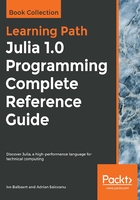
functions
Functions can be nested, as demonstrated in the following example:
function a(x) z = x * 2 function b(z) z += 1 end b(z) end d = 5 a(d) #=> 11
A function can also be recursive, that is, it can call itself. To show some examples, we need to be able to test a condition in code. The simplest way to do this in Julia is to use the ternary operator ? of the form expr ? b : c (ternary because it takes three arguments). Julia also has a normal if construct. (Refer to the Conditional evaluation section of Chapter 4, Control Flow.) expr is a condition and, if it is true, then b is evaluated and the value is returned, else c is evaluated. This is used in the following recursive definition to calculate the sum of all the integers up to and including a certain number:
sum(n) = n > 1 ? sum(n-1) + n : n
The recursion ends because there is a base case: when n is 1, this value is returned. Here is the famous function to calculate the nth Fibonacci number that is defined as the sum of the two previous Fibonacci numbers:
fib(n) = n < 2 ? n : fib(n-1) + fib(n-2)
When using recursion, care should be taken to define a base case to stop the calculation. Also, although Julia can nest very deeply, watch out for stack overflows, because, until now, Julia has not done tail call optimization automatically.