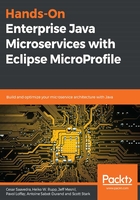
The Health Check Java API
Most of the plumbing is performed by the application framework that implements the MP-HC specification. Your part is to decide how liveness or readiness are determined through the health check procedures that your microservice defines using the MP-HC API.
To do this, you need to implement a health check procedure by implementing one or more instances of the HealthCheck interface using beans that are marked with a Health annotation.
The HealthCheck interface is provided in the following code block:
package org.eclipse.microprofile.health;
@FunctionalInterface
public interface HealthCheck {
HealthCheckResponse call();
}
The code for the Health annotation is provided in the following code block:
package org.eclipse.microprofile.health;
import javax.inject.Qualifier;
import java.lang.annotation.Documented;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Qualifier
@Documented
@Retention(RetentionPolicy.RUNTIME)
public @interface Health {
}
An example HealthCheck implementation that represents the status of a hypothetical disk space check is shown in the following example. Note that the check includes the current free space as part of the response data. The HealthCheckResponse class supports a builder interface to populate the response object.
Following is a hypothetical disk space HealthCheck procedure implementation:
import javax.enterprise.context.ApplicationScoped;
import org.eclipse.microprofile.health.Health;
import org.eclipse.microprofile.health.HealthCheck;
import org.eclipse.microprofile.health.HealthCheckResponse;
@Health
@ApplicationScoped
public class CheckDiskspace implements HealthCheck {
@Override
public HealthCheckResponse call() {
return HealthCheckResponse.named("diskspace")
.withData("free", "780mb")
.up()
.build();
}
}
In this example, we created a health response that is named diskspace with a status of up and custom data named free with a string value of 780mb.
Another health check example representing some service endpoint is shown in the following.
A hypothetical service HealthCheck procedure implementation is shown here:
package io.packt.hc.rest;
//ServiceCheck example
import javax.enterprise.context.ApplicationScoped;
import org.eclipse.microprofile.health.Health;
import org.eclipse.microprofile.health.HealthCheck;
import org.eclipse.microprofile.health.HealthCheckResponse;
@Health
@ApplicationScoped
public class ServiceCheck implements HealthCheck {
public HealthCheckResponse call() {
return HealthCheckResponse.named("service-check")
.withData("port", 12345)
.withData("isSecure", true)
.withData("hostname", "service.jboss.com")
.up()
.build();
}
}
In this example, we created a health response named service-check with a status of up that includes the following additional data:
- A port item with an integer value of 12345
- An isSecure item with a Boolean value of true
- A hostname item with a string value of service.jboss.com
The CDI-managed health checks are discovered and registered automatically by the application runtime. The runtime automatically exposes an HTTP endpoint, /health, used by the cloud platform to poke into your application to determine its state. You can test this by building the Chapter04-healthcheck application and running it. You will see the following output:
Scotts-iMacPro:hc starksm$ mvn package
[INFO] Scanning for projects…
...
Resolving 144 out of 420 artifacts
[INFO] Repackaging .war: /Users/starksm/Dev/JBoss/Microprofile/PacktBook/Chapter04-metricsandhc/hc/target/health-check.war
[INFO] Repackaged .war: /Users/starksm/Dev/JBoss/Microprofile/PacktBook/Chapter04-metricsandhc/hc/target/health-check.war
[INFO] -----------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] -----------------------------------------------------------------------
[INFO] Total time: 7.660 s
[INFO] Finished at: 2019-04-16T21:55:14-07:00
[INFO] -----------------------------------------------------------------------
Scotts-iMacPro:hc starksm$ java -jar target/health-check-thorntail.jar
2019-04-16 21:57:03,305 INFO [org.wildfly.swarm] (main) THORN0013: Installed fraction: MicroProfile Fault Tolerance - STABLE io.thorntail:microprofile-fault-tolerance:2.4.0.Final
…
2019-04-16 21:57:07,449 INFO [org.jboss.as.server] (main) WFLYSRV0010: Deployed "health-check.war" (runtime-name : "health-check.war")
2019-04-16 21:57:07,453 INFO [org.wildfly.swarm] (main) THORN99999: Thorntail is Ready
Once the server has started, test the health checks by querying the health endpoint:
Scotts-iMacPro:Microprofile starksm$ curl -s http://localhost:8080/health | jq
{
"outcome": "UP",
"checks": [
{
"name": "service-check",
"state": "UP",
"data": {
"hostname": "service.jboss.com",
"port": 12345,
"isSecure": true
}
},
{
"name": "diskspace",
"state": "UP",
"data": {
"free": "780mb"
}
}
]
}
This shows the overall health to be UP. The overall status is the logical OR of all of the health check procedures found in the application. In this case, it is AND of the two health check procedures we have seen: diskspace and service-check.