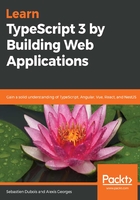
Installing dependencies with npm and package.json
As we have explained, if you have package.json, then you can define both dependencies and devDependencies. Here's an example:
{
"name": "npm-install-example",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "dSebastien <seb@dsebastien.net>
(https://www.dsebastien.net)",
"dependencies": {
"lodash": "4.17.11"
},
"devDependencies": {
"karma": "3.0.0"
}
}
In this example, we have defined the dependencies section and we have added a dependency in relation to the 4.17.11 version of the lodash (https://lodash.com) library. In devDependencies, we have defined a dependency in relation to the karma (https://karma-runner.github.io) test runner.
Once your dependencies are configured, you can invoke npm install to install them. Here's the output of that command for the preceding example:
$ npm install
...
npm WARN npm-install-example@1.0.0 No description
npm WARN npm-install-example@1.0.0 No repository field.
npm WARN npm-install-example@1.0.0 No license field.
npm WARN optional SKIPPING OPTIONAL DEPENDENCY: fsevents@1.2.4 (node_modules\fsevents):
npm WARN notsup SKIPPING OPTIONAL DEPENDENCY: Unsupported platform for fsevents@1.2.4: wanted
{"os":"darwin","arch":"any"} (current: {"os":"win32","arch":"x64"})
added 257 packages from 502 contributors and audited 2342 packages in 14.476s
found 0 vulnerabilities
Ignore the warnings for now and concentrate on the last two lines as those are the interesting bits:
- The installer has installed our dependencies.
- The installer did not find any known security vulnerabilities.
If you try this out, you'll see that you now have a new folder called node_modules. Go ahead and give it a look; verify that you do find lodash and karma in it, along with many other dependencies.
One huge benefit of this approach is that you can, of course, version the package.json file with the rest of your project. Along with that, you can also be sure that the other developers working on the same project will easily be able to install everything they need to start working on it.