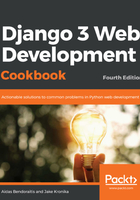
How it works...
The example of Idea will generate a model that is similar to the following:
class Idea(models.Model):
title_bg = models.CharField(
_("Title (Bulgarian)"),
max_length=200,
)
title_hr = models.CharField(
_("Title (Croatian)"),
max_length=200,
)
# titles for other languages…
title_sv = models.CharField(
_("Title (Swedish)"),
max_length=200,
)
content_bg = MultilingualTextField(
_("Content (Bulgarian)"),
)
content_hr = MultilingualTextField(
_("Content (Croatian)"),
)
# content for other languages…
content_sv = MultilingualTextField(
_("Content (Swedish)"),
)
class Meta:
verbose_name = _("Idea")
verbose_name_plural = _("Ideas")
def __str__(self):
return self.title
If there were any language codes with a dash, like "de-ch" for Swiss German, the fields for those languages would be replaced with underscores, like title_de_ch and content_de_ch.
In addition to the generated language-specific fields, there will be two properties – title and content – that will return the corresponding field in the currently active language. These will fall back to the default language if no localized field content is available.
The MultilingualCharField and MultilingualTextField fields will juggle the model fields dynamically, depending on your LANGUAGES setting. They will overwrite the contribute_to_class() method that is used when the Django framework creates the model classes. The multilingual fields dynamically add character or text fields for each language of the project. You'll need to create a database migration to add the appropriate fields in the database. Also, the properties are created to return the translated value of the currently active language or the main language, by default.
In the administration, get_multilingual_field_names() will return a list of language-specific field names, starting with one of the default languages and then proceeding with the other languages from the LANGUAGES setting.
Here are a couple of examples of how you might use the multilingual fields in templates and views.
If you have the following code in the template, it will show the text in the currently active language, let's say Lithuanian, and will fall back to English if the translation doesn't exist:
<h1>{{ idea.title }}</h1>
<div>{{ idea.content|urlize|linebreaks }}</div>
If you want to have your QuerySet ordered by the translated titles, you can define it as follows:
>>> lang_code = input("Enter language code: ")
>>> lang_code_underscored = lang_code.replace("-", "_")
>>> qs = Idea.objects.order_by(f"title_{lang_code_underscored}")