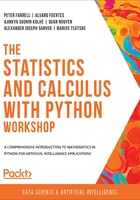
Control Flow Methods
Control flow is a general term that denotes any programming syntax that can redirect the execution of a program. Control flow methods in general are what allow programs to be dynamic in their execution and computation: depending on the current state of a program or its input, the execution of that program and thus its output will dynamically change.
if Statements
The most common form of control flow in any programming language is conditionals, or if statements. if statements are used to check for a specific condition about the current state of the program and, depending on the result (whether the condition is true or false), the program will execute different sets of instructions.
In Python, the syntax of an if statement is as follows:
if [condition to check]:
[instruction set to execute if condition is true]
Given the readability of Python, you can probably already guess how conditionals work: when the execution of a given program reaches a conditional and checks the condition in the if statement, if the condition is true, the indented set of instructions inside the if statement will be executed; otherwise, the program will simply skip those instructions and move on.
Within an if statement, it is possible for us to check for a composite condition, which is a combination of multiple inpidual conditions. For example, using the and keyword, the following if block is executed when both of its conditions are satisfied:
if [condition 1] and [condition 2]:
[instruction set]
Instead of doing this, we can use the or keyword in a composite condition, which will display positive (true) if either the condition to the left or to the right of the keyword is true. It is also possible to keep extending a composite condition with more than one and/or keyword to implement conditionals that are nested on multiple levels.
When a condition is not satisfied, we might want our program to execute a different set of instructions. To implement this logic, we can use elif and else statements, which should immediately follow an if statement. If the condition in the if statement is not met, our program will move on and evaluate the subsequent conditions in the elif statements; if none of the conditions are met, any code inside an else block will be executed. An if...elif...else block in Python is in the following form:
if [condition 1]:
[instruction set 1]
elif [condition 2]:
[instruction set 2]
...
elif [condition n]:
[instruction set n]
else:
[instruction set n + 1]
This control flow method is very valuable when there is a set of possibilities that our program needs to check for. Depending on which possibility is true at a given moment, the program should execute the corresponding instructions.
Exercise 1.01: Divisibility with Conditionals
In mathematics, the analysis of variables and their content is very common, and one of the most common analyses is the pisibility of an integer. In this exercise, we will use if statements to consider the pisibility of a given number by 5, 6, or 7.
Perform the following steps in order to achieve this:
- Create a new Jupyter notebook and declare a variable named x whose value is any integer, as shown in the following code:
x = 130
- After that declaration, write an if statement to check whether x is pisible by 5 or not. The corresponding code block should print out a statement indicating whether the condition has been met:
if x % 5 == 0:
print('x is pisible by 5')
Here, % is the modulo operator in Python; the var % n expression returns the remainder when we pide the var variable by the number, n.
- In the same code cell, write two elif statements to check whether x is pisible by 6 and 7, respectively. Appropriate print statements should be placed under their corresponding conditionals:
elif x % 6 == 0:
print('x is pisible by 6')
elif x % 7 == 0:
print('x is pisible by 7')
- Write the final else statement to print out a message stating that x is not pisible by either 5, 6, or 7 (in the same code cell):
else:
print('x is not pisible by 5, 6, or 7')
- Run the program with a different value assigned to x each time to test the conditional logic we have. The following output is an example of this with x assigned with the value 104832:
x is pisible by 6
- Now, instead of printing out a message about the pisibility of x, we would like to write that message to a text file. Specifically, we want to create a file named output.txt that will contain the same message that we printed out previously.
To do this, we can use the with keyword together with the open() function to interact with the text file. Note that the open() function takes in two arguments: the name of the file to write to, which is output.txt in our case, and w (for write), which specifies that we would like to write to file, as opposed to reading the content from a file:
if x % 5 == 0:
with open('output.txt', 'w') as f:
f.write('x is pisible by 5')
elif x % 6 == 0:
with open('output.txt', 'w') as f:
f.write('x is pisible by 6')
elif x % 7 == 0:
with open('output.txt', 'w') as f:
f.write('x is pisible by 7')
else:
with open('output.txt', 'w') as f:
f.write('x is not pisible by 5, 6, or 7')
- Check the message in the output text file for its correctness. If the x variable still holds the value of 104832, your text file should have the following contents:
x is pisible by 6
In this exercise, we applied the usage of conditionals to write a program that determines the pisibility of a given number by 6, 3, and 2 using the % operator. We also saw how to write content to a text file in Python. In the next section, we will start discussing loops in Python.
Note
The code lines in the elif block are executed sequentially, and breaks from sequence, when any one of the conditions is true. This implies that when x is assigned the value 30, once x%5==0 is satisfied, x%6==0 is not checked.
To access the source code for this specific section, please refer to https://packt.live/3dNflxO.
You can also run this example online at https://packt.live/2AsqO8w.
Loops
Another widely used control flow method is the use of loops. These are used to execute the same set of instructions repeatedly over a specified range or while a condition is met. There are two types of loops in Python: while loops and for loops. Let's understand each one in detail.
The while Loop
A while loop, just like an if statement, checks for a specified condition to determine whether the execution of a given program should keep on looping or not. For example, consider the following code:
>>> x = 0
>>> while x < 3:
... print(x)
... x += 1
0
1
2
In the preceding code, after x was initialized with the value 0, a while loop was used to successively print out the value of the variable and increment the same variable at each iteration. As you can imagine, when this program executes, 0, 1, and 2 will be printed out and when x reaches 3, the condition specified in the while loop is no longer met, and the loop therefore ends.
Note that the x += 1 command corresponds to x = x + 1, which increments the value of x during each iteration of the loop. If we remove this command, then we would get an infinite loop printing 0 each time.
The for Loop
A for loop, on the other hand, is typically used to iterate through a specific sequence of values. Using the range function in Python, the following code produces the exact same output that we had previously:
>>> for x in range(3):
... print(x)
0
1
2
The in keyword is the key to any for loop in Python: when it is used, the variable in front of it will be assigned values inside the iterator that we'd like to loop through sequentially. In the preceding case, the x variable is assigned the values inside the range(3) iterator—which are, in order, 0, 1, and 2—at each iteration of the for loop.
Instead of range(), other types of iterators can also be used in a Python for loop. The following table gives a brief summary of some of the most common iterators to be used in for loops. Don't worry if you are not familiar with the data structures included in this table; we will cover those concepts later in this chapter:

Figure 1.1: List of datasets and their examples
It is also possible to nest multiple loops inside one another. While the execution of a given program is inside a loop, we can use the break keyword to exit the current loop and move on with the execution.
Exercise 1.02: Number Guessing Game
For this exercise, we will put our knowledge of loops to practice and write a simple guessing game. A target integer between 0 and 100 is randomly selected at the beginning of the program. Then, the program will take in user inputs as guesses of what this number is. In response, the program will print out a message saying Lower if the guess is greater than the actual target, or Higher if the opposite is true. The program should terminate when the user guesses correctly.
Perform the following steps to complete this exercise:
- In the first cell of a new Jupyter notebook, import the random module in Python and use its randint function to generate random numbers:
import random
true_value = random.randint(0, 100)
Every time the randint() function is called, it generates a random integer between the two numbers passed to it; in our case, an integer between 0 and 100 will be generated.
While they are not needed for the rest of this exercise, if you are curious about other functionalities that the random module offers, you can take a look at its official documentation at https://docs.python.org/3/library/random.html.
Note
The rest of the program should also be put in the current code cell.
- Use the input() function in Python to take in the user's input and assign the returned value to a variable (guess, in the following code). This value will be interpreted as the guess of what the target is from the user:
guess = input('Enter your guess: ')
- Convert the user input into an integer using the int() function and check it against the true target. Print out appropriate messages for all possible cases of the comparison:
guess = int(guess)
if guess == true_value:
print('Congratulations! You guessed correctly.')
elif guess > true_value:
print('Lower.') # user guessed too high
else:
print('Higher.') # user guessed too low
Note
The # symbol in the code snippet below denotes a code comment. Comments are added into code to help explain specific bits of logic.
- With our current code, the int() function will throw an error and crash the entire program if its input cannot be converted into an integer (for example, when the input is a string character). For this reason, we need to implement the code we have inside a try...except block to handle the situation where the user enters a non-numeric value:
try:
if guess == true_value:
print('Congratulations! You guessed correctly.')
elif guess > true_value:
print('Lower.') # user guessed too high
else:
print('Higher.') # user guessed too low
# when the input is invalid
except ValueError:
print('Please enter a valid number.')
- As of now, the user can only guess exactly once before the program terminates. To implement the feature that would allow the user to repeatedly guess until they find the target, we will wrap the logic we have developed so far in a while loop, which will break if and only if the user guesses correctly (implemented by a while True loop with the break keyword placed appropriately).
The complete program should look similar to the following code:
import random
true_value = random.randint(0, 100)
while True:
guess = input('Enter your guess: ')
try:
guess = int(guess)
if guess == true_value:
print('Congratulations! You guessed correctly.')
break
elif guess > true_value:
print('Lower.') # user guessed too high
else:
print('Higher.') # user guessed too low
# when the input is invalid
except ValueError:
print('Please enter a valid number.')
- Try rerunning the program by executing the code cell and test out different input options to ensure that the program can process its instructions nicely, as well as handle cases of invalid inputs. For example, the output the program might produce when the target number is randomly selected to be 13 is as follows:
Enter your guess: 50
Lower.
Enter your guess: 25
Lower.
Enter your guess: 13
Congratulations! You guessed correctly.
In this exercise, we have practiced using a while loop in a number guessing game to solidify our understanding of the usage of loops in programming. In addition, you have been introduced to a method of reading in user input and the random module in Python.
Note
To access the source code for this specific section, please refer to https://packt.live/2BYK6CR.
You can also run this example online at https://packt.live/2CVFbTu.
Next, we will start considering common Python data structures.