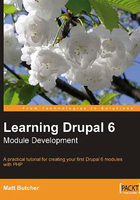
Finishing Touches: hook_help()
We now have a functioning module. However, there is one last thing that a good Drupal module should have. Modules should implement the hook_help()
function to provide help text for module users.
Our module is not very complex. Our help hook won't be, either:
/** * Implementation of hook_help() */ function goodreads_help($path, $arg) { if ($path == 'admin/help#goodreads') { $txt = 'The Goodreads module uses the !goodreads_url XML ' .'API to retrieve a list of books and display it as block ' .'content.'; $link = l('Goodreads.com', 'http://www.goodreads.com'); $replace = array( '!goodreads_url' => $link ); return '<p>'. t($txt, $replace) .'</p>'; } }
The hook_help()
function gets two parameters: $path
, which contains a URI fragment indicating what help page was called, and $arg
, which might contain extra information.
In a complex instance of hook_help()
, you might use a switch statement on the $path
, returning different help text for each possible path. For our module, only one path is likely to be passed in: the path to general help in the administration section. This path follows the convention admin/help#<module name>
, where<module name>
should be replaced with the name of the module (e.g. goodreads)
.
The function is straightforward: The help text is stored in the $txt
variable. If our module required any additional setup or was linked to additional help, we would want to indicate this here. But in our case, all we really need is a basic description of what the module does.
We also want to insert a link back to Goodreads.com. To do this, we create the placeholder (!goodreads_url
) in the $txt
content, and then create the link ($link
) by calling the l()
function.
Since we are going to pass the $txt
string and the link into the translation function, and let that function replace the placeholder with the link, we need to put $link
inside an array—the $replace
array.
Finally the help text is returned after being passed through the t()
translation function.
This help text will now be accessible on the Administer | Help page:

That is all there is to creating help text.
It is good coding practice to include help text with your module. And the more complex a module is, the better the help text should be. In future chapters, we will start every module by implementing hook_help()
. Often, though, we will keep text in the help function brief for the sake of keeping the book concise and readable.
In your own modules you may want to create more verbose help text. This is helpful to users who don't have access to the code (or to a book explaining how the module works in detail).