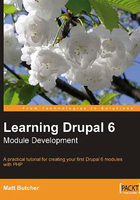
Starting Out
Our first module is going to fetch XML data from Goodreads (http://www.goodreads.com), a free social networking site for avid readers. There, users track the books they are reading and have read, rate books and write reviews, and share their reading lists with friends.
Reading lists at Goodreads are stored in bookshelves. These bookshelves are accessible over a web-based XML/RSS API. We will use that API to display a reading list on the Philosopher Bios website we introduced in Chapter 1.
To integrate the Goodreads information in Drupal, we will create a small module. Since this is our first module, we will get into greater details, since they will be commonplace in the later chapters.
A Place for the Module
In Drupal, every module is contained in its own directory. This simplifies organization; all of the module's files are located in one place.
To keep naming consistent throughout the module (a standard in Drupal), we will name our directory with the module name. Later, we will install this module in Drupal, but for development, the module directory can be wherever it is most convenient.
Once we have created a directory named goodreads
, we can start creating files for our module. The first file we need to create is the .info
(dot-info) file.
Creating a .info File
Before we start coding our new module, we need to create a simple text file that will hold some basic information about our module. Various Drupal components use the information in this file for module management.
The .info
file is written as a PHP INI file, which is a simple configuration file format.
Note
If you are interested in the details of INI file processing, you can visit http://php.net/manual/en/function.parse-ini-file.php for a description of this format and how it can be parsed in PHP.
Our .info
file will only be five lines long, which is probably about average.
The .info
file must follow the standard naming conventions for modules. It must be named<modulename>.info
, where<modulename>
is the same as the directory name. Our file, then, will be called goodreads.info
.
Following are the contents of goodreads.info:
;$Id$ name = "Goodreads Bookshelf" description = "Displays items from a Goodreads Bookshelf" core = 6.x php = 5.1
This file isn't particularly daunting. The first line of the file is, at first glance, the most cryptic. However, its function is mundane: it is a placeholder for Drupal's CVS server.
Drupal, along with its modules, is maintained on a central CVS (Concurrent Version System) server. CVS is a version control system. It tracks revisions to code over time. One of its features is its ability to dynamically insert version information into a file. However, it needs to know where to insert the information. The placeholder for this is the special string $Id$
. But since this string isn't actually a directive in the .info
file, it is commented out with the PHP INI comment character,;
(semi-colon).
The next four directives each provide module information to Drupal.
The name
directive provides a human-readable display name for the module. In the last chapter, we briefly discussed the Drupal module installation and configuration interface. The names of the modules we saw there were extracted from the name
directive in their corresponding .info
files. Here's an example:

In this above screenshot, the names Aggregator and Blog are taken from the values of the name
directives in these modules' .info
files.
While making the module's proper name short and concise is good (as we did when naming the module directory goodreads
above), the display name should be helpful to the user. That usually means that it should be a little longer, and a little more descriptive.
However, there is no need to jam all of the module information into the name
directive. The description
directive is a good place for providing a sentence or two describing the module's function and capabilities.
The third directive is the core
directive.
This directive specifies what version of Drupal is required for this module to function properly. Our value, 6.x
, indicates that this module will run on Drupal 6 (including its minor revisions). In many cases, the Drupal packager will be able to automatically set this (correctly). But Drupal developers are suggesting that this directive be set manually for those who work from CVS.
Finally, the php
directive makes it possible to specify a minimum version number requirement for PHP. PHP 5, for example, has many features that are missing in PHP 4 (and the modules in this book make use of such features). For that reason, we explicitly note that our modules require at least PHP version 5.1.
That's all there is to our first module .info
file. In later chapters, we will see some other possible directives. But what we have here is sufficient for our Goodreads module.
Now, we are ready to write some PHP code.