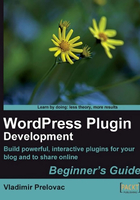
Time for action – Create a 'Hello World!' widget
As usual, we will start building our plugin with the necessary plugin information.
Later, we will create a function to display Hello World text and then register that function as a widget.
- Create a new folder called
wp-wall
. - Create a new
wp-wall.php
file. Insert the following plugin information:/* Plugin Name: WP Wall Version: 0.1 Description: "Wall" widget that appears in your blog's side barUsers can add a quick comment and it will appear in the sidebar immediately (without reloading the page). Author: Vladimir Prelovac Author URI: http://www.prelovac.com/vladimir Plugin URI: http://www.prelovac.com/vladimir/wordpress-plugins/wp-wall */ global $wp_version; $exit_msg='WP Wall requires WordPress 2.6 or newer. <a href="http://codex.wordpress.org/Upgrading_WordPress">Please update!</a>'; if (version_compare($wp_version,"2.3","<")) { exit ($exit_msg); }
- Add a variable that will hold the path to our plugin. We will use it later.
$wp_wall_plugin_url = trailingslashit( WP_PLUGIN_URL.'/'.dirname( plugin_basename(__FILE__) );
- Add the function for displaying the text:
function WPWall_Widget() { echo "Hello World!"; }
- Register our function as a widget. We do this by hooking to
init
action and usingregister_sidebar_widget
call:function WPWall_Init() { // register widget register_sidebar_widget('WP Wall', 'WPWall_Widget'); } add_action('init', 'WPWall_Init'); ?>
- Now upload and enable our plugin. Our widget will appear on the widgets page of the administrative panel. In order to make it active, you need to drag and drop it onto the sidebar.
- Visit your site and you will notice the Hello World! text printed on your sidebar. I know it is not pretty yet, but we just wanted to get it out there for now.
What just happened?
The action hook init
executes just after WordPress has finished loading. It is a good choice for inserting general plugin initialization code such as registering widgets. We use it to insert our init
function:
add_action('init', 'WPWall_Init');
The widget is registered in WordPress with register_sidebar_widget()
taking the widget name and the callback function responsible for drawing the widget.
function WPWall_Init() { // register widget register_sidebar_widget('WP Wall', 'WPWall_Widget'); }
In our case, the callback function simply prints out the Hello World! text on the screen.
function WPWall_Widget() { echo "Hello World!"; }
Register widgets with description
The official WordPress documentation encourages the use of the register_sidebar_widget()
function for compatibility reasons, instead of the more powerful wp_register_sidebar_widget()
.
The latter function allows us to enter the widget description, shown on the widgets screen.
Alternatively, you can use this code to register widget:
$widget_optionss = array('classname' => 'WPWall_Widget', 'description' => "A comments 'Wall' for your sidebar." ); wp_register_sidebar_widget('WPWall_Widget', 'WP Wall', 'WPWall_Widget', $widget_options);
Which shows a description for the widget:

Note
Quick reference
Init
: Action best used for initializing plugin data ad registering widgets.
register_sidebar_widget($name, $callback)
: Registers a widget with a name and a callback function parameter. More information is available at: http://automattic.com/code/widgets/plugins/
wp_register_sidebar_widget($id, $name, $callback, $options)
: A more powerful way to register widgets allowing us to specify the description in the options.