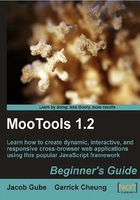
Time for action—rewriting our script unobtrusively
Let's rewrite the previous example to follow unobtrusive JavaScript principles:
- First, we'll remove all the
onclick
attributes. By doing so, we've effectively separated our website's functionality from its content structure. Here's the revised code:<html> <head> <script type="text/javascript"> function mouseClick() { alert( 'You clicked me!' ); } </script> </head> <body> <ul id="nav"> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Contact</a></li> </ul> </body> </html>
- Now remove the
mouseClick()
function; we don't need it anymore.<html> <head> <!-- mouseClick() function is now bye-bye. --> </head> <body> <ul id="nav"> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Contact</a></li> </ul> </body>
- Include the MooTools framework and put our code inside
window.addEvent('domready')
.<html> <head> <script type="text/javascript" src="mootools-1.2.1-core-nc.js"> </script> <script type="text/javascript"> window.addEvent('domready', function() { // Our code will go in here }); </script> </head> <body> <ul id="nav"> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Contact</a></li> </ul> </body> </html>
- Add an event listener to all child links of
<ul id="nav">
. You'll learn more about event listeners in Chapter 5.<html> <head> <script type="text/javascript" src="mootools-1.2.1-core-nc.js"> </script> <script type="text/javascript"> window.addEvent('domready', function() { $$('#nav a').addEvent('click', function() { // The alert function will go in here }); }); </script> </head> <body> <ul id="nav"> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Contact</a></li> </ul> </body> </html>
- Put our
alert()
function back, inside theaddEvent
method.<html> <head> <script type="text/javascript" src="mootools-1.2.1-core-nc.js"> </script> <script type="text/javascript"> window.addEvent('domready', function() { $$('#nav a').addEvent('click', function() { alert( 'You clicked me!' ); }); }); </script> </head> <body> <ul id="nav"> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Contact</a></li> </ul> </body> </html>
- Save your work and open it in a web browser. Click on any link. It should open up a dialog box just like in our obtrusive example.
Just like before, if you did it correctly, you should see a dialog box that says You clicked me!
What just happened?
What we did was fix our poorly-written and obtrusive JavaScript example by rewriting our code without the use of inline event handlers. We leaned on MooTools to help us do this effortlessly by using the $$()
function to select all<a>
elements inside our<ul id="nav">
element and then added an unobtrusive click
event listener/handler.
Now we can go onto explore key aspects of what we just did.
Removing our inline event handlers
The first step we did was to remove all of our onclick
attributes. This not only makes our HTML and JavaScript leaner and cleaner, but we also effectively separated our web page's structure from its functionality. Now, whenever we want to add, remove, or edit our event handler for clicks, we just have to do it in one place—MooTools code.
Using the DOM to handle events
In step 4, we leveraged the DOM to add events to our<a>
elements, first matching all elements in the DOM that match our criteria via the $$()
function, namely all<a>
elements that are the children of<ul id="nav">
element, which translates to $$('#nav a')
(notice that we selected it using CSS selectors syntax). Then we call the MooTools Element method, .addEvent()
, gave it a click
argument. The .addEvent()
method with a click
argument, in plain English, just says: add an event handler to perform this function when a 'click' event is triggered.
function() { alert( 'You clicked me!' ); }
Now, when we clicked on any link on our web page, the alert()
function was triggered and we saw a dialog box.
You'll learn plenty more about selecting DOM elements and adding event handlers in Chapters 3 and 5 respectively. For now, suffice it to say that we were able to revolutionize our "obtrusive" JavaScript code into something magnitudes better. Now, if we wanted to later on remove the event handler, we just remove it from our MooTools script, instead of going to multiple pages to remove the inline event handler.
Pop quiz — — rewriting our script unobstrusively
Which of the following statements is wrong?
- Unobtrusive JavaScript separates JavaScript from your content's structure.
- Unobtrusive JavaScript makes updating scripts easier.
- Unobtrusive JavaScript is exclusive to the MooTools framework.
- Unobtrusive JavaScript, oftentimes, can reduce the amount of code you have to write.
Alright, I think you've had more than your fair share of unobtrusive-ness, but if you want to learn more, check out this URL: http://www.onlinetools.org/articles/unobtrusivejavascript/.
Now it's time to move onto another topic: MooTools classes.