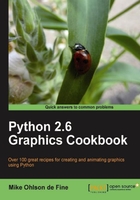
Draw three overlapping rectangles by changing the numerical values defining their position, shape, and color variables.
As before the instructions used in recipe 1 should be used.
Just use the name 3rectangles.py
when you write, save, and execute this program.
# 3rectangles.py #>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> from Tkinter import * root = Tk() root.title('Overlapping rectangles') cw = 240 # canvas width ch = 180 # canvas height canvas_1 = Canvas(root, width=cw, height=200, background="green") canvas_1.grid(row=0, column=1) # dark blue rectangle - painted first therefore at the bottom x_start = 10 y_start = 30 x_width =70 y_height = 90 kula ="darkblue" canvas_1.create_rectangle( x_start, y_start,\ x_start + x_width, y_start + y_height, fill=kula) # dark red rectangle - second therefore in the middle x_start = 30 y_start = 50 kula ="darkred" canvas_1.create_rectangle( x_start, y_start,\ x_start + x_width, y_start + y_height, fill=kula) # dark green rectangle - painted last therefore on top of previous # ones. x_start = 50 y_start = 70 kula ="darkgreen" canvas_1.create_rectangle( x_start, y_start,\ x_start + x_width, y_start + y_height, fill=kula) #>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
The results are given in the next screenshot, which shows overlapping rectangles drawn in sequence.

The height and width of the rectangles have been kept the same but their start positions have been shifted to different positions. In addition a common-named variable called kula
has been used as a common attribute in each create-rectangle()
method. In between drawing each rectangle a new value is assigned to kula
to give each successive rectangle a different color.
Just a short comment on color here. Ultimately colors used in Tkinter code are number values with each numerical value specifying how much red, green, and blue to mix together. However, inside the Tkinter libraries are collections of romantically named colors like 'rose pink', 'lime green', and 'cornflower blue'. Each named color is assigned a specific numerical value that creates the color suggested by the name. Sometimes you will see some of these referred to as web colors. Sometimes you assign a name to a color only to have the Python interpreter reject it as unrecognized or use only shades of grey. This tricky topic is sorted out in Chapter 5, The Magic of Color.
The way the attributes of drawn shapes have been specified may appear to be long winded. The programs would be shorter and neater if we just put the absolute numerical values of the parameters inside the methods that draw the functions. In the preceding example, we could have expressed the rectangles as:
canvas_1.create_rectangle( 10, 30, 70 ,90, , fill='darkblue') canvas_1.create_rectangle( 30, 50, 70 ,90, , fill='darkred') canvas_1.create_rectangle( 50, 70, 70 ,90, , fill='darkgreen')
There are good reasons for specifying attribute values outside of the methods.
- It allows you to make reusable code that can be used repeatedly regardless of specific values of variables.
- It makes the code self-explanatory when you use
x_start
instead of a number. - It lets you change the values of attributes in a controlled systematic manner. There are many examples of this later.