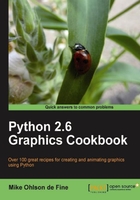
Here we attempt to execute a Tkinter command inside the Python program. The Tkinter instruction will create a canvas and then draw a straight line on it.
- In a text editor, type the code given below.
- Save this as a file named
simple_line_1.py
, inside the directory calledconstr
again. - As before open up an X terminal or DOS window if you are using MS Windows.
- Change directory into
constr
- wheresimple_line_1.py
is located. - Type
python simple_line_1.py
and your program should execute. The command terminal result should look like the following screenshot: - The Tkinter canvas output should look like the following screenshot:
- This proves that your Python interpreter works, your editor works, and the Tkinter module works. This is not a trivial achievement – you are definitely ready for great things. Well done.
#>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>> from Tkinter import * root = Tk() root.title('Very simple Tkinter line') canvas_1 = Canvas(root, width=300, height=200, background="#ffffff") canvas_1.grid(row=0, column=0) canvas_1.create_line(10,20 , 50,70) root.mainloop() #>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
To draw a line, we only need to give the start point and the end point.
The start point is the first pair of numbers in canvas_1.create_line(10,20 , 50,70)
.In another way, the start is given by the coordinates x_start=10
and y_start=20
. The end point of the line is specified by the second pair of numbers x_end=50
and y_end=70
. The units of measurement are pixels. A pixel is the smallest dot that can be displayed on our screen.
For all other properties like line thickness or color, default values of the create_line()
method are used.
However, should you want to change color or thickness, you just do it by specifying the settings.