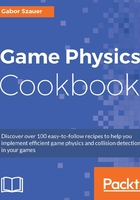
Scaling
The scale of a matrix is stored in the main diagonal of the matrix. The scale is stored as a vec3
. Each element of the vec3
represents the scale on the corresponding axis. Row and Column major matrices store scale information in the same elements:

The interesting thing with storing scale inside a matrix is that it shares some of the same elements as the rotation part of the matrix. Because of this, extracting the scale of a matrix may not always yield the numbers you would expect.
Getting ready
We're going to implement three functions. One function will retrieve the scale stored inside a matrix. The other two will return a new matrix, containing only the specified scale.
How to do it…
Follow these steps to set and retrieve the scale of a matrix:
- Add the declaration for all scaling functions to
matrices.h
:mat4 Scale(float x, float y, float z); mat4 Scale(const vec3& vec); vec3 GetScale(const mat4& mat);
- Implement the functions that create a 4 X 4 matrix out of scaling information in
matrices.cpp
:mat4 Scale(float x, float y, float z) { return mat4( x, 0.0f, 0.0f, 0.0f, 0.0f, y, 0.0f, 0.0f, 0.0f, 0.0f, z, 0.0f, 0.0f, 0.0f, 0.0f, 1.0f ); } mat4 Scale(const vec3&vec) { return mat4( vec.x, 0.0f, 0.0f, 0.0f, 0.0f, vec.y,0.0f, 0.0f, 0.0f, 0.0f, vec.z,0.0f, 0.0f, 0.0f, 0.0f, 1.0f ); }
- Implement the function to retrieve scaling from a 4 X 4 matrix in
matrices.cpp
.vec3 GetScale(const mat4& mat) { return vec3(mat._11, mat._22, mat._33); }
How it works…
Both of the Scale
functions create a new matrix by placing the scaling values into the main diagonal of the identity matrix. The GetScale
function retrieves the main diagonal of the matrix packed into a vec3
. If a matrix contains scale and rotation information, the result of the GetScale
function might not be what you expect.