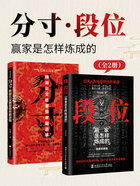
上QQ阅读APP看本书,新人免费读10天
设备和账号都新为新人
pre(预定义格式文本)
HTML <pre> 元素表示预定义格式文本。在该元素中的文本通常按照原文件中的编排,以等宽字体的形式展现出来,文本中的空白符(比如空格和换行符)都会显示出来。(紧跟在 <pre> 开始标签后的换行符也会被省略)
复杂的例子:
1. Plain text rendering - This is a simple text block in <pre> format. - All spaces, tabs, and newlines are preserved. 2. Code snippet for (int i = 0; i < 10; i++) { Console.WriteLine($"Value: {i}"); } 3. Tables with spaces for alignment Name Age Country John Doe 25 USA Alice Smith 30 UK 张伟 35 中国 4. ASCII art _______ / \ | O O | | ^ | <-- A simple face! \_______/ 5. Special characters - HTML entities: <, >, &, " - Unicode: ✔, ✘, ☺, ☀, ✈, 🧑💻 6. Mixed languages English: Hello, World! 中文: 你好,世界! 日本語: こんにちは、世界! Español: ¡Hola, Mundo! 7. Wide characters ┌───────────┬────────────┐ │ Header │ Values │ ├───────────┼────────────┤ │ Data A │ 1234567890 │ │ 数据 B │ 测试文本 │ └───────────┴────────────┘ 8. Nested tags (escaped) - <b>Bold</b> - <i>Italic</i> - <a href='example.com'>Link</a> 9. Indentation and tabs Root ├── Child 1 │ ├── Subchild 1.1 │ └── Subchild 1.2 └── Child 2 └── Subchild 2.1
简单多行代码:
from flux.api import ImageRequest # this will create an api request directly but not block until the generation is finished request = ImageRequest("A beautiful beach", name="flux.1.1-pro") # or: request = ImageRequest("A beautiful beach", name="flux.1.1-pro", api_key="your_key_here") # any of the following will block until the generation is finished request.url # -> https:<...>/sample.jpg request.bytes # -> b"..." bytes for the generated image request.save("outputs/api.jpg") # saves the sample to local storage request.image # -> a PIL image
单行的预定义格式:
cd $HOME && git clone https://github.com/black-forest-labs/flux
C#排序算法,测试多行代码下的排版表现:
using System; class Program { static void Main(string[] args) { int[] array = { 34, 7, 23, 32, 5, 62 }; Console.WriteLine("Original array:"); PrintArray(array); QuickSort(array, 0, array.Length - 1); Console.WriteLine("Sorted array:"); PrintArray(array); } static void QuickSort(int[] array, int left, int right) { if (left < right) { int pivotIndex = Partition(array, left, right); QuickSort(array, left, pivotIndex - 1); // Sort left part QuickSort(array, pivotIndex + 1, right); // Sort right part } } static int Partition(int[] array, int left, int right) { int pivot = array[right]; int i = left - 1; for (int j = left; j < right; j++) { if (array[j] <= pivot) { i++; Swap(array, i, j); } } Swap(array, i + 1, right); return i + 1; } static void Swap(int[] array, int i, int j) { int temp = array[i]; array[i] = array[j]; array[j] = temp; } static void PrintArray(int[] array) { Console.WriteLine(string.Join(", ", array)); } }
未格式化的JavaScript代码,没有缩进或换行,所有代码压缩为一行:
function bubbleSort(arr){for(let i=0;i<arr.length;i++){for(let j=0;j<arr.length-i-1;j++){if(arr[j]>arr[j+1]){let temp=arr[j];arr[j]=arr[j+1];arr[j+1]=temp;}}}return arr;}let array=[5,3,8,4,2];console.log("Original Array:",array);console.log("Sorted Array:",bubbleSort(array));