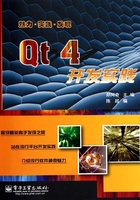
5.5 消息对话框:QMessageBox类
在实际的程序开发中,经常会用到各种各样的消息框来给用户一些提示或提醒,Qt提供了QMessageBox类来实现此项功能。
常用的消息对话框包括Question消息框、Information消息框、Warning消息框、Critical消息框、About(关于)消息框、About(关于)Qt消息框以及Custom(自定义)消息框。其中,Question消息框、Information消息框、Warning消息框和Critical消息框的用法大同小异,这些消息框一般都包含为用户提供一些提醒或一些简单询问的一个图标、一条提示信息以及若干个按钮。Question消息框为正常的操作提供一个简单的询问;Information消息框为正常的操作提供一个提示;Warning消息框提醒用户发生了一个错误;Critical消息框警告用户发生了一个严重错误。
下面的例子演示了各种消息对话框的使用。首先是完成界面的设计,具体实现步骤如下:
(1) 添加该工程的提供主要显示标准消息对话框界面的函数所在的文件,在“DialogExample”项目名上单击鼠标右键,在弹出的快捷菜单中选择“Add New...”菜单项,在弹出的对话框中选择“C++ Class”选项,单击“OK”按钮,弹出“C++ Class Wizard”对话框,在Base class后面的下拉列表框中输入基类名“QDialog”,在Class name后面的文本框中输入类的名称“MsgBoxDlg”。
(2) 单击“Next”按钮,单击“Finish”按钮,在该工程中就添加了msgboxdlg.h头文件和msgboxdlg.cpp源文件。
(3) 打开“msgboxdlg.h”头文件,完成所需要的各种控件和完成功能的槽函数的声明,具体代码如下:
//添加的头文件 #include <QLabel> #include <QPushButton> #include <QGridLayout> class MsgBoxDlg : public QDialog { Q_OBJECT public: MsgBoxDlg(); private slots: void showQuestionMsg(); void showInformationMsg(); void showWarningMsg(); void showCriticalMsg(); void showAboutMsg(); void showAboutQtMsg(); private: QLabel *label; QPushButton *questionBtn; QPushButton *informationBtn; QPushButton *warningBtn; QPushButton *criticalBtn; QPushButton *aboutBtn; QPushButton *aboutQtBtn; QGridLayout *mainLayout; };
(4) 打开“msgboxdlg.cpp”文件,完成所需要的各种控件的创建和完成槽函数的实现,具体代码如下:
MsgBoxDlg::MsgBoxDlg() { setWindowTitle(tr("标准消息对话框的实例")); //设置对话框的标题 label =new QLabel; label->setText(tr("请选择一种消息框")); questionBtn =new QPushButton; questionBtn->setText(tr("QuestionMsg")); informationBtn =new QPushButton; informationBtn->setText(tr("InformationMsg")); warningBtn =new QPushButton; warningBtn->setText(tr("WarningMsg")); criticalBtn =new QPushButton; criticalBtn->setText(tr("CriticalMsg")); aboutBtn =new QPushButton; aboutBtn->setText(tr("AboutMsg")); aboutQtBtn =new QPushButton; aboutQtBtn->setText(tr("AboutQtMsg")); //布局 mainLayout =new QGridLayout(this); mainLayout->addWidget(label,0,0,1,2); mainLayout->addWidget(questionBtn,1,0); mainLayout->addWidget(informationBtn,1,1); mainLayout->addWidget(warningBtn,2,0); mainLayout->addWidget(criticalBtn,2,1); mainLayout->addWidget(aboutBtn,3,0); mainLayout->addWidget(aboutQtBtn,3,1); //事件关联 connect(questionBtn,SIGNAL(clicked()),this,SLOT(showQuestionMsg())); connect(informationBtn,SIGNAL(clicked()),this,SLOT(showInformationM sg())); connect(warningBtn,SIGNAL(clicked()),this,SLOT(showWarningMsg())); connect(criticalBtn,SIGNAL(clicked()),this,SLOT(showCriticalMsg())); connect(aboutBtn,SIGNAL(clicked()),this,SLOT(showAboutMsg())); connect(aboutQtBtn,SIGNAL(clicked()),this,SLOT(showAboutQtMsg())); } void MsgBoxDlg::showQuestionMsg() { } void MsgBoxDlg::showInformationMsg() { } void MsgBoxDlg::showWarningMsg() { } void MsgBoxDlg::showCriticalMsg() { } void MsgBoxDlg::showAboutMsg() { } void MsgBoxDlg::showAboutQtMsg() { }
下面是完成主对话框的操作过程:
(1) 在dialog.h中,添加头文件:
#include "msgboxdlg.h"
添加private成员变量如下:
QPushButton *MsgBtn;
添加实现各种消息对话框实例的MsgBoxDlg类:
MsgBoxDlg *msgDlg;
(2) 添加private slots槽函数:
void showMsgDlg();
(3) 在dialog.cpp中,构造函数中的代码如下:
MsgBtn =new QPushButton; //创建控件对象 MsgBtn->setText(tr("标准消息对话框实例"));
添加布局管理:
mainLayout->addWidget(MsgBtn,3,1);
最后添加事件关联:
connect(MsgBtn,SIGNAL(clicked()),this,SLOT(showMsgDlg()));
其中,槽函数showMsgDlg()的实现代码如下:
void Dialog::showMsgDlg() { msgDlg =new MsgBoxDlg(); msgDlg->show(); }
(4) 运行该程序后,单击“标准消息对话框实例”按钮后,显示效果如图5.6所示。
5.5.1 Question消息框
Question消息框使用QMessageBox::question()函数完成,此函数形式如下:
StandardButton QMessageBox::question ( QWidget* parent, //消息框的父窗口指针 const QString& title, //消息框的标题栏 const QString& text, //消息框的文字提示信息 StandardButtons buttons=Ok, //注(1) StandardButton defaultButton=NoButton //注(2) );
注:1.填写希望在消息框中出现的按钮,可根据需要在标准按钮中选择,用"|"连写,默认为QMessageBox::Ok。QMessageBox类提供了许多标准按钮,比如QMessageBox::Ok、QMessageBox::Close、QMessageBox::Discard等等。虽然在此可以任意选择,但并不是随意选择的,应注意按常规成对出现,比如,一般Save与Discard成对出现,而Abort、Retry、Ignore一起出现。
2.默认按钮,即消息框出现时,焦点默认处于哪个按钮上。
完成文件msgboxdlg.cpp中的槽函数showQuestionMsg()的具体代码如下:
void MsgBoxDlg::showQuestionMsg() { label->setText(tr("Question Message Box")); switch(QMessageBox::question(this,tr("Question消息框"), tr("您现在已经修改完成,是否要结束程序?"), QMessageBox::Ok|QMessageBox::Cancel,QMessageBox::Ok)) { case QMessageBox::Ok: label->setText("Question button/Ok"); break; case QMessageBox::Cancel: label->setText("Question button/Cancel"); break; default: break; } return; }
在msgboxdlg.cpp的开头添加头文件:
#include<QMessageBox>
运行程序,单击“QuestionMsg”按钮后,显示效果如图5.6(a)所示。
5.5.2 Information消息框
Information消息框使用QMessageBox::information()函数完成,函数形式如下:
StandardButton QMessageBox::information ( QWidget*parent, //消息框的父窗口指针 const QString& title, //消息框的标题栏 const QString& text, //消息框的文字提示信息 StandardButtons buttons=Ok, //同上Question消息框的注释内容 StandardButton defaultButton=NoButton //同上Question消息框的注释内容 );
完成文件msgboxdlg.cpp中的槽函数showInformationMsg(),具体实现如下:
void MsgBoxDlg::showInformationMsg() { label->setText(tr("Information Message Box")); QMessageBox::information(this,tr("Information消息框"), tr("这是Information消息框测试,欢迎您!")); return; }
运行程序,单击“InformationMsg”按钮后,显示效果如图5.6(b)所示。
5.5.3 Warning消息框
Warning消息框使用QMessageBox::warning()函数完成,函数形式如下:
StandardButton QMessageBox::warning ( QWidget* parent, //消息框的父窗口指针 const QString& title, //消息框的标题栏 const QString& text, //消息框的文字提示信息 StandardButtons buttons=Ok, //同上Question消息框的注释内容 StandardButton defaultButton=NoButton //同上Question消息框的注释内容 );
完成文件msgboxdlg.cpp中的槽函数showWarningMsg(),具体实现如下:
void MsgBoxDlg::showWarningMsg() { label->setText(tr("Warning Message Box")); switch(QMessageBox::warning(this,tr("Warning消息框"), tr("您修改的内容还未保存,是否要保存对文档的修改?"), QMessageBox::Save|QMessageBox::Discard|QMessageBox::Cancel, QMessageBox::Save)) { case QMessageBox::Save: label->setText(tr("Warning button/Save")); break; case QMessageBox::Discard: label->setText(tr("Warning button/Discard")); break; case QMessageBox::Cancel: label->setText(tr("Warning button/Cancel")); break; default: break; } return; }
运行程序,单击“WarningMsg”按钮后,显示效果如图5.6(c)所示。
5.5.4 Critical消息框
Critical消息框使用QMessageBox::critical()函数完成,函数形式如下:
StandardButton QMessageBox::critical ( QWidget* parent, //消息框的父窗口指针 const QString& title, //消息框的标题栏 const QString& text, //消息框的文字提示信息 StandardButtons buttons=Ok, //同上Question消息框的注释内容 StandardButton defaultButton=NoButton //同上Question消息框的注释内容 );
完成文件msgboxdlg.cpp中的槽函数showCriticalMsg()的具体实现如下:
void MsgBoxDlg::showCriticalMsg() { label->setText(tr("Critical Message Box")); QMessageBox::critical(this,tr("Critical消息框"),tr("这是一个Critical消 息框测试!")); return; }
运行程序,单击“CriticalMsg”按钮后,显示效果如图5.6(d)所示。
5.5.5 About消息框
About消息框使用QMessageBox::about()函数完成,函数形式如下:
void QMessageBox::about ( QWidget* parent, //消息框的父窗口指针 const QString& title, //消息框的标题栏 const QString& text //消息框的文字提示信息 );
完成文件msgboxdlg.cpp中的槽函数showAboutMsg()的具体实现如下:
void MsgBoxDlg::showAboutMsg() { label->setText(tr("About Message Box")); QMessageBox::about(this,tr("About消息框"),tr("这是一个About消息框测试!")); return; }
运行程序,单击“AboutMsg”按钮后,显示效果如图5.6(e)所示。
5.5.6 About Qt消息框
About Qt消息框使用QMessageBox:: aboutQt ()函数完成,函数形式如下:
void QMessageBox::aboutQt ( QWidget* parent, //消息框的父窗口指针 const QString& title=QString() //消息框的标题栏 );
完成文件msgboxdlg.cpp中的槽函数showAboutQtMsg(),具体实现如下:
void MsgBoxDlg::showAboutQtMsg() { label->setText(tr("About Qt Message Box")); QMessageBox::aboutQt(this,tr("About Qt消息框")); return; }
运行程序,单击“AboutQtMsg”按钮后,显示效果如图5.6(f)所示。